Last active
September 17, 2018 13:55
-
-
Save mikeumus/e94b57fab07d5c49f20479d34a09b812 to your computer and use it in GitHub Desktop.
WiP - Find a value and the deep path to it in a object.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
function pullKeys(obj, results, path) { | |
var breadcrumb = path; | |
for (var prop in obj) { | |
if (obj.hasOwnProperty(prop)) { | |
// path = breadcrumb ? breadcrumb ? path : prop : path; | |
// console.log('\n\n path: \n', path, '\n\n'); | |
if (typeof obj[prop] === "object") { | |
path = path ? breadcrumb ? breadcrumb += `.${prop}` : path += `.${prop}` : prop; | |
pullKeys(obj[prop], results, path); | |
} else { | |
var innerObj = {}; | |
innerObj[path] = obj[prop]; | |
results.push(innerObj); | |
} | |
path = breadcrumb ? breadcrumb : path; | |
console.log('\n\n path: \n', path, '\n\n'); | |
} | |
} | |
// console.log('\n\n breadcrumb: \n', breadcrumb, '\n\n'); | |
path = ''; | |
} | |
function deepFind(results, target) { | |
var found = []; | |
results.forEach( function(item) { | |
if ( Object.values(item)[0] === target ) { | |
found.push(item); | |
} | |
}); | |
return found; | |
} | |
function deepSearch(obj, target) { | |
var results = []; | |
pullKeys(obj, results); | |
return deepFind(results, target); | |
} | |
var testObj1 = { | |
notAnObject: 'just a string', | |
iamObject: { now: 'what?' } | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Diagram visualizing some of the recursion happening here:
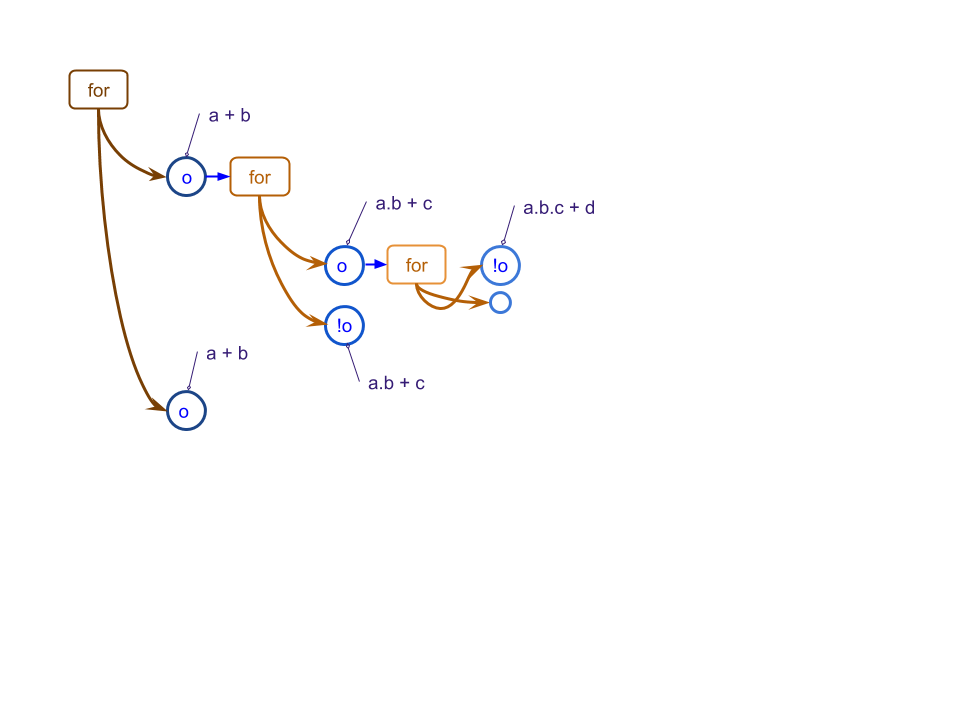
https://docs.google.com/drawings/d/1YKj-CEq---aRj7mQFYbYz99qwiRy4fzC481td0OsU-4/edit?usp=sharing